
Introduction to jQuery
jQuery has revolutionized the way web developers approach JavaScript programming. It's a powerful library that simplifies complex tasks, making web development more efficient and enjoyable. Whether you're a beginner or an experienced developer, understanding jQuery is essential for building modern, interactive websites.
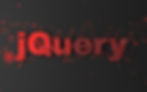
Understanding the Basics of jQuery
Selectors and Filters
One of the fundamental concepts of jQuery is selectors. Selectors allow you to target HTML elements based on their tag name, class, ID, or attributes. Filters, on the other hand, enable you to refine your selection further.
DOM Manipulation
jQuery makes it easy to manipulate the Document Object Model (DOM) of a web page. You can add, remove, or modify elements dynamically, enhancing the user experience without reloading the entire page.
Events Handling
Handling events is a crucial aspect of web development, and jQuery simplifies this process with its intuitive event handling methods. You can easily attach event listeners to HTML elements and execute custom functions when events occur.
Advanced jQuery Techniques
Animation and Effects
jQuery provides built-in methods for creating stunning animations and effects. Whether you want to fade elements in and out, slide them up or down, or create custom animations, jQuery has you covered.
AJAX with jQuery
Asynchronous JavaScript and XML (AJAX) is a powerful technique for building dynamic web applications, and jQuery simplifies AJAX interactions with its `$.ajax()` method. You can fetch data from a server and update parts of a web page without refreshing the entire page.
Plugins and Extensions
One of the strengths of jQuery is its vast ecosystem of plugins and extensions. These plugins extend the functionality of jQuery, allowing you to add advanced features such as sliders, carousels, form validation, and more to your websites with ease.
Benefits of Using jQuery
Simplified Syntax
jQuery's concise and intuitive syntax allows you to accomplish complex tasks with minimal code. It abstracts away many of the complexities of JavaScript, making it accessible to developers of all skill levels.
Cross-browser Compatibility
One of the biggest headaches for web developers is ensuring compatibility across different browsers. jQuery takes care of this for you, handling browser quirks and inconsistencies behind the scenes, so you can focus on building great websites.
Extensive Plugin Ecosystem
With thousands of plugins available, jQuery enables you to add a wide range of features to your websites without reinventing the wheel. Whether you need a date picker, a lightbox gallery, or a form validation plugin, chances are there's a jQuery plugin that does exactly what you need.
How to Use jQuery in Web Development
Integration
To start using jQuery in your web projects, simply include the jQuery library in your HTML file using the `<script>` tag. You can either download the jQuery library and host it locally or link to a CDN-hosted version for improved performance and caching.
Document Ready Function
It's essential to ensure that your jQuery code executes only after the DOM has fully loaded. To achieve this, wrap your jQuery code inside the `$(document).ready()` function.
Selectors
jQuery provides powerful selectors that allow you to target and manipulate HTML elements based on their attributes, classes, IDs, and hierarchy.
Event Handling
jQuery simplifies event handling by providing methods to attach event listeners to HTML elements and execute custom functions when events occur.
Animation
jQuery includes built-in methods for creating smooth animations and effects, allowing you to enhance the user experience with visually appealing transitions.
Best Practices for jQuery Development
Code Optimization
Optimizing your jQuery code is essential for improving performance and maintainability. Minify your JavaScript files, avoid unnecessary DOM manipulations, and cache jQuery selectors for better performance.
Unobtrusive JavaScript
Follow the principle of unobtrusive JavaScript, separating behavior from markup. Keep your HTML clean and semantic, and use JavaScript to enhance functionality rather than cluttering your markup with inline event handlers.
Progressive Enhancement
Embrace the philosophy of progressive enhancement, starting with a solid foundation of semantic HTML and enhancing it with JavaScript and CSS. This ensures that your website remains accessible and functional even for users with JavaScript disabled.
Common Mistakes to Avoid
Overusing jQuery
While jQuery is a powerful tool, it's essential not to overuse it. Evaluate whether jQuery is necessary for each task and consider native JavaScript alternatives where appropriate.
Ignoring Performance Considerations
jQuery can sometimes introduce performance overhead, especially when dealing with large-scale applications. Be mindful of performance considerations and optimize your code accordingly.
Not Understanding Callbacks
Callbacks are a fundamental concept in JavaScript and jQuery, allowing you to execute code asynchronously. Make sure you understand how callbacks work and how to use them effectively in your jQuery code.
Conclusion
jQuery is a versatile and powerful tool that simplifies JavaScript development, enhances cross-browser compatibility, and accelerates the creation of dynamic web applications. By mastering jQuery's syntax and features, developers can create more engaging and interactive user experiences while reducing development time and complexity.
FAQs
1. Is jQuery still relevant in modern web development?
Yes, jQuery is still widely used in web development, especially for its ease of use and cross-browser compatibility.
2. Are there any alternatives to jQuery?
Yes, there are alternatives such as React, Vue.js, and Angular, but jQuery remains a popular choice for its simplicity and versatility.
3. Can I use jQuery with other JavaScript frameworks?
Yes, jQuery can be used alongside other JavaScript frameworks, although you may encounter some compatibility issues.
4. Is jQuery necessary for building responsive websites?
While jQuery can be helpful for adding interactive elements to responsive websites, it's not strictly necessary. Responsive design can be achieved using CSS media queries and JavaScript.
5. Where can I learn more about jQuery?
You can find comprehensive documentation and tutorials on the official jQuery website, as well as numerous online resources and courses.